Auto Reload is Spring Boot using Dev Tools and Live-Reload
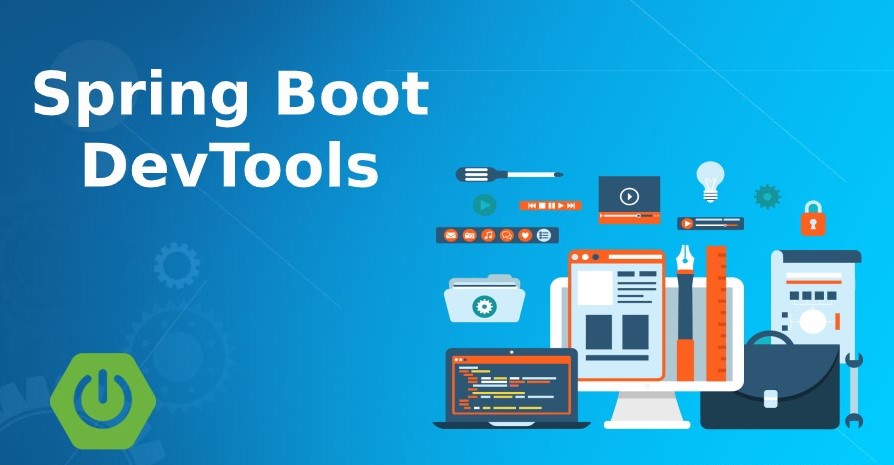
Live Reload feature is used to reflect the changes on browser automatically on code change , this is a Huge Time Saver for any developer. In Spring boot to do that we use Spring dev tools. Internally its a web socket communication from web server to browser , for node js or javascript grant or gulp are present in order to perform live reload.
Steps To Setup
Dev Tools
Add the dependency spring-boot-devtools to your project’s build file i.e. Gradle or Maven
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
Live Reload
Install LiveReload extension for your browser (Usually Chrome is preferred)
- go to app store https://chrome.google.com/webstore/category/extensions
-
select Live Reload and install and Enable it.
- Restart your Spring Boot application.
Usage
- Now on code change your code and on browser automatically things would start to reflect on Browser.
Troubleshooting
Sometimes there are issues in case you are in Intellij
- Make sure to go to settings > Compiler > Build project Automatically
- make sure that this checkbox is ticked.
- Double shift and go to registry
- Search for compiler.automake.allow.when.app.running and mark it as “checked”
Customizations
- Make sure if you are using Thymeleaf to switch caching off by adding following line in application.properties file
- Dev tools automatically does that , however in case you are facing the issue, do add it.
spring.thymeleaf.cache=false
- add specific path , understand that this refers to the package from componentscan root folder
- This will make sure that auto live reload runs only when you are working in these package
spring.devtools.restart.additional-paths = /path_To_Folder
- Similarly to exclude any folder
spring.devtools.exclude = /path_of_Excluded_Folder
- To Disable live-Reload , update following property in application.properties file
- Especially in the UAT or Prod environments
- its always advisable to remove this dependency all together.
spring.devtools.livereload.enabled=false
Conclusion
Dev tools are very powerful developer tools that can not only help us in live Reload however also for “Remote Debug Tunneling” - for docker containers and “Remote Update and Restart” pushing changes to remote.